这篇文章主要为大家详细介绍了HttpHelper类的使用方法,HttpHelper类及调用,具有一定的参考价值,感兴趣的小伙伴们可以参考一下
本文实例为大家分享了HttpHelper类的方法使用,供大家参考,具体内容如下文章源自设计学徒自学网-https://www.sx1c.com/25860.html
首先列出HttpHelper类文章源自设计学徒自学网-https://www.sx1c.com/25860.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 | /// <summary> /// Http操作类 /// </summary> public class HttpHelper { private static log4net.ILog mLog = log4net.LogManager.GetLogger( "HttpHelper" ); [DllImport( "wininet.dll" , CharSet = CharSet.Auto, SetLastError = true )] public static extern bool InternetSetCookie( string lpszUrlName, string lbszCookieName, string lpszCookieData); [DllImport( "wininet.dll" , CharSet = CharSet.Auto, SetLastError = true )] public static extern bool InternetGetCookie( string lpszUrlName, string lbszCookieName, StringBuilder lpszCookieData, ref int lpdwSize); public static StreamReader mLastResponseStream = null ; public static System.IO.StreamReader LastResponseStream { get { return mLastResponseStream; } } private static CookieContainer mCookie = null ; public static CookieContainer Cookie { get { return mCookie; } set { mCookie = value; } } private static CookieContainer mLastCookie = null ; public static HttpWebRequest CreateWebRequest( string url, HttpRequestType httpType, string contentType, string data, Encoding requestEncoding, int timeout, bool keepAlive) { if (String.IsNullOrWhiteSpace(url)) { throw new Exception( "URL为空" ); } HttpWebRequest webRequest = null ; Stream requestStream = null ; byte [] datas = null ; switch (httpType) { case HttpRequestType.GET: case HttpRequestType.DELETE: if (!String.IsNullOrWhiteSpace(data)) { if (!url.Contains( '?' )) { url += "?" + data; } else url += "&" + data; } if (url.StartsWith( "https:" )) { System.Net.ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls11 | SecurityProtocolType.Tls12; ServicePointManager.ServerCertificateValidationCallback = new System.Net.Security.RemoteCertificateValidationCallback(CheckValidationResult); } webRequest = (HttpWebRequest)WebRequest.Create(url); webRequest.Method = Enum.GetName( typeof (HttpRequestType), httpType); if (contentType != null ) { webRequest.ContentType = contentType; } if (mCookie == null ) { webRequest.CookieContainer = new CookieContainer(); } else { webRequest.CookieContainer = mCookie; } if (keepAlive) { webRequest.KeepAlive = keepAlive; webRequest.ReadWriteTimeout = timeout; webRequest.Timeout = 60000; mLog.Info( "请求超时时间..." + timeout); } break ; default : if (url.StartsWith( "https:" )) { System.Net.ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls11 | SecurityProtocolType.Tls12; ServicePointManager.ServerCertificateValidationCallback = new System.Net.Security.RemoteCertificateValidationCallback(CheckValidationResult); } webRequest = (HttpWebRequest)WebRequest.Create(url); webRequest.Method = Enum.GetName( typeof (HttpRequestType), httpType); if (contentType != null ) { webRequest.ContentType = contentType; } if (mCookie == null ) { webRequest.CookieContainer = new CookieContainer(); } else { webRequest.CookieContainer = mCookie; } if (keepAlive) { webRequest.KeepAlive = keepAlive; webRequest.ReadWriteTimeout = timeout; webRequest.Timeout = 60000; mLog.Info( "请求超时时间..." + timeout); } if (!String.IsNullOrWhiteSpace(data)) { datas = requestEncoding.GetBytes(data); } if (datas != null ) { webRequest.ContentLength = datas.Length; requestStream = webRequest.GetRequestStream(); requestStream.Write(datas, 0, datas.Length); requestStream.Flush(); requestStream.Close(); } break ; } //mLog.InfoFormat("请求 Url:{0},HttpRequestType:{1},contentType:{2},data:{3}", url, Enum.GetName(typeof(HttpRequestType), httpType), contentType, data); return webRequest; } public static CookieContainer GetLastCookie() { return mLastCookie; } /// <summary> /// 设置HTTP的Cookie,以后发送和请求用此Cookie /// </summary> /// <param name="cookie">CookieContainer</param> public static void SetHttpCookie(CookieContainer cookie) { mCookie = cookie; } private static HttpWebRequest mLastAsyncRequest = null ; public static HttpWebRequest LastAsyncRequest { get { return mLastAsyncRequest; } set { mLastAsyncRequest = value; } } /// <summary> /// 发送请求 /// </summary> /// <param name="url">请求Url</param> /// <param name="httpType">请求类型</param> /// <param name="contentType">contentType:application/x-www-form-urlencoded</param> /// <param name="data">请求数据</param> /// <param name="encoding">请求数据传输时编码格式</param> /// <returns>返回请求结果</returns> public static string SendRequest( string url, HttpRequestType httpType, string contentType, string data, Encoding requestEncoding, Encoding reponseEncoding, params AsyncCallback[] callBack) { int timeout = 0; bool keepAlive = false ; if (callBack != null && callBack.Length > 0 && callBack[0] != null ) { keepAlive = true ; timeout = 1000*60*60; mLog.Info( "写入读取超时时间..." + timeout); } // mLog.Info("开始创建请求...."); HttpWebRequest webRequest = CreateWebRequest(url, httpType, contentType, data, requestEncoding,timeout,keepAlive); string ret = null ; // mLog.Info("创建请求结束...."); if (callBack != null && callBack.Length > 0 && callBack[0] != null ) { // mLog.Info("开始异步请求...."); mLastAsyncRequest = webRequest; webRequest.BeginGetResponse(callBack[0], webRequest); } else { // mLog.Info("开始同步请求...."); StreamReader sr = new StreamReader(webRequest.GetResponse().GetResponseStream(), reponseEncoding); ret = sr.ReadToEnd(); sr.Close(); } mLastCookie = webRequest.CookieContainer; //mLog.InfoFormat("结束请求 Url:{0},HttpRequestType:{1},contentType:{2},结果:{3}", url, Enum.GetName(typeof(HttpRequestType), httpType), contentType,ret); return ret; } /// <summary> /// Http上传文件 /// </summary> public static string HttpUploadFile( string url, string path) { using (FileStream fs = new FileStream(path, FileMode.Open, FileAccess.Read)) { // 设置参数 HttpWebRequest request = WebRequest.Create(url) as HttpWebRequest; CookieContainer cookieContainer = new CookieContainer(); request.CookieContainer = cookieContainer; request.AllowAutoRedirect = true ; request.AllowWriteStreamBuffering = false ; request.SendChunked = true ; request.Method = "POST" ; request.Timeout = 300000; string boundary = DateTime.Now.Ticks.ToString( "X" ); // 随机分隔线 request.ContentType = "multipart/form-data;charset=utf-8;boundary=" + boundary; byte [] itemBoundaryBytes = Encoding.UTF8.GetBytes( "\r\n--" + boundary + "\r\n" ); byte [] endBoundaryBytes = Encoding.UTF8.GetBytes( "\r\n--" + boundary + "--\r\n" ); int pos = path.LastIndexOf( "\\" ); string fileName = path.Substring(pos + 1); //请求头部信息 StringBuilder sbHeader = new StringBuilder( string .Format( "Content-Disposition:form-data;name=\"file\";filename=\"{0}\"\r\nContent-Type:application/octet-stream\r\n\r\n" , fileName)); byte [] postHeaderBytes = Encoding.UTF8.GetBytes(sbHeader.ToString()); request.ContentLength = itemBoundaryBytes.Length + postHeaderBytes.Length + fs.Length + endBoundaryBytes.Length; using (Stream postStream = request.GetRequestStream()) { postStream.Write(itemBoundaryBytes, 0, itemBoundaryBytes.Length); postStream.Write(postHeaderBytes, 0, postHeaderBytes.Length); int bytesRead = 0; int arrayLeng = fs.Length <= 4096 ? ( int )fs.Length : 4096; byte [] bArr = new byte [arrayLeng]; int counter = 0; while ((bytesRead = fs.Read(bArr, 0, arrayLeng)) != 0) { counter++; postStream.Write(bArr, 0, bytesRead); } postStream.Write(endBoundaryBytes, 0, endBoundaryBytes.Length); } //发送请求并获取相应回应数据 using (HttpWebResponse response = request.GetResponse() as HttpWebResponse) { //直到request.GetResponse()程序才开始向目标网页发送Post请求 using (Stream instream = response.GetResponseStream()) { StreamReader sr = new StreamReader(instream, Encoding.UTF8); //返回结果网页(html)代码 string content = sr.ReadToEnd(); return content; } } } } public static string HttpUploadFile( string url, MemoryStream files, string fileName) { using (MemoryStream fs = files) { // 设置参数 HttpWebRequest request = WebRequest.Create(url) as HttpWebRequest; CookieContainer cookieContainer = new CookieContainer(); request.CookieContainer = cookieContainer; request.AllowAutoRedirect = true ; request.AllowWriteStreamBuffering = false ; request.SendChunked = true ; request.Method = "POST" ; request.Timeout = 300000; string boundary = DateTime.Now.Ticks.ToString( "X" ); // 随机分隔线 request.ContentType = "multipart/form-data;charset=utf-8;boundary=" + boundary; byte [] itemBoundaryBytes = Encoding.UTF8.GetBytes( "\r\n--" + boundary + "\r\n" ); byte [] endBoundaryBytes = Encoding.UTF8.GetBytes( "\r\n--" + boundary + "--\r\n" ); //请求头部信息 StringBuilder sbHeader = new StringBuilder( string .Format( "Content-Disposition:form-data;name=\"file\";filename=\"{0}\"\r\nContent-Type:application/octet-stream\r\n\r\n" , fileName)); byte [] postHeaderBytes = Encoding.UTF8.GetBytes(sbHeader.ToString()); request.ContentLength = itemBoundaryBytes.Length + postHeaderBytes.Length + fs.Length + endBoundaryBytes.Length; using (Stream postStream = request.GetRequestStream()) { postStream.Write(itemBoundaryBytes, 0, itemBoundaryBytes.Length); postStream.Write(postHeaderBytes, 0, postHeaderBytes.Length); int bytesRead = 0; int arrayLeng = fs.Length <= 4096 ? ( int )fs.Length : 4096; byte [] bArr = new byte [arrayLeng]; int counter = 0; fs.Position = 0; while ((bytesRead = fs.Read(bArr, 0, arrayLeng)) != 0) { counter++; postStream.Write(bArr, 0, bytesRead); } postStream.Write(endBoundaryBytes, 0, endBoundaryBytes.Length); } //发送请求并获取相应回应数据 using (HttpWebResponse response = request.GetResponse() as HttpWebResponse) { //直到request.GetResponse()程序才开始向目标网页发送Post请求 using (Stream instream = response.GetResponseStream()) { StreamReader sr = new StreamReader(instream, Encoding.UTF8); //返回结果网页(html)代码 string content = sr.ReadToEnd(); return content; } } } } #region public static 方法 /// <summary> /// 将请求的流转化为字符串 /// </summary> /// <param name="info"></param> /// <returns></returns> public static string GetStr(Stream info) { string result = "" ; try { using (StreamReader sr = new StreamReader(info, System.Text.Encoding.UTF8)) { result = sr.ReadToEnd(); sr.Close(); } } catch { } return result; } /// <summary> /// 参数转码 /// </summary> /// <param name="str"></param> /// <returns></returns> public static string stringDecode( string str) { return HttpUtility.UrlDecode(HttpUtility.UrlDecode(str, System.Text.Encoding.GetEncoding( "UTF-8" )), System.Text.Encoding.GetEncoding( "UTF-8" )); } /// <summary> /// json反序列化 /// </summary> /// <typeparam name="T"></typeparam> /// <param name="json"></param> /// <returns></returns> public static T Deserialize<T>( string json) { try { T obj = Activator.CreateInstance<T>(); using (MemoryStream ms = new MemoryStream(System.Text.Encoding.UTF8.GetBytes(json))) { DataContractJsonSerializer serializer = new DataContractJsonSerializer(obj.GetType()); return (T)serializer.ReadObject(ms); } } catch { return default (T); } } #endregion public static bool CheckValidationResult( object sender, X509Certificate certificate, X509Chain chain, SslPolicyErrors sslPolicyErrors) { // 总是接受 return true ; } } public enum HttpRequestType { POST, GET, DELETE, PUT, PATCH, HEAD, TRACE, OPTIONS } |
然后列出HttpHelper的调用文章源自设计学徒自学网-https://www.sx1c.com/25860.html
1、不带参数调用文章源自设计学徒自学网-https://www.sx1c.com/25860.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | public bool ConnectServer() { try { string url = "https://i.cnblogs.com" ; string xml = HttpHelper.SendRequest(url, HttpRequestType.POST, null , null , Encoding.UTF8, Encoding.UTF8); NormalResponse nr = HuaweiXMLHelper.GetNormalResponse(xml); if (nr.Code == "0" ) { HttpHelper.SetHttpCookie(HttpHelper.GetLastCookie()); mIsConnect = true ; return true ; } else { mIsConnect = false ; return false ; } } catch (System.Exception ex) { mIsConnect = false ; return false ; } } |
2.带参数调用文章源自设计学徒自学网-https://www.sx1c.com/25860.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | private bool HandleIntelligentTask( string taskId, bool bStop) { try { if (!mIsConnect) { return false ; } StringBuilder sb = new StringBuilder(); sb.AppendFormat( "<request>\r\n" ); sb.AppendFormat( "<task_id>{0}</task_id>\r\n" , taskId); //<!-- task-id为调用方生成的UUID或其它串 --> sb.AppendFormat( "<status>{0}</status>\r\n" ,bStop?0:1); sb.AppendFormat( "</request>\r\n" ); string xml = sb.ToString(); string url = mIAServerUrl + "/sdk_service/rest/video-analysis/handle-intelligent-analysis" ; string xml2 = HttpHelper.SendRequest(url, HttpRequestType.POST, "text/plain;charset=utf-8" , xml, Encoding.UTF8, Encoding.UTF8); NormalResponse nr = HuaweiXMLHelper.GetNormalResponse(xml2); if (nr.Code == "0" ) { return true ; } else { return false ; } } catch (System.Exception ex) { return false ; } } |
3.异步调用文章源自设计学徒自学网-https://www.sx1c.com/25860.html
文章源自设计学徒自学网-https://www.sx1c.com/25860.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 | private void ReStartAlarmServer(List< string > list, string alarmUrl, Thread[] listThread) { StopAlarm(alarmUrl, listThread); listThread[0]= new Thread( new ThreadStart( delegate () { try { if (!mIsConnect) { mLog.Error( "未登录!--ReStartAlarmServer-结束!" ); return ; } mLog.Info( "ReStartAlarmServer开始报警连接...." ); if (String.IsNullOrWhiteSpace(alarmUrl)) return ; mLog.InfoFormat( "ReStartAlarmServer请求报警:URL={0}" , alarmUrl); string xml = "task-id=0" ; string xml2 = HttpHelper.SendRequest(alarmUrl, HttpRequestType.POST, "application/x-www-form-urlencoded" , xml, Encoding.UTF8, Encoding.UTF8, AlarmCallBack); mLog.Info( "ReStartAlarmServer报警连接成功!" ); } catch (System.Threading.ThreadAbortException ex) { mLog.Info( "ReStartAlarmServer线程已人为终止!" + ex.Message, ex); } catch (System.Exception ex) { mLog.Error( "ReStartAlarmServer开始报警连接失败:" + ex.Message, ex); mLog.Info( "ReStartAlarmServer开始重新报警连接...." ); mTimes = 50; } finally { } })); listThread[0].IsBackground = true ; listThread[0].Start(); } private void AlarmCallBack(IAsyncResult ir) { try { HttpWebRequest webRequest = (HttpWebRequest)ir.AsyncState; string salarmUrl = webRequest.Address.OriginalString; Thread[] alarmThead = dicAlarmUrls[salarmUrl]; HttpWebResponse response = (HttpWebResponse)webRequest.EndGetResponse(ir); Stream stream = response.GetResponseStream(); alarmThead[1]= new Thread( new ThreadStart( delegate () { try { byte [] buffer = new byte [mAlarmReadCount]; int count = 0; string strMsg = "" ; int startIndex = -1; int endIndex = -1; NormalResponse res = null ; DateTime dtStart = DateTime.Now; DateTime dtEnd = DateTime.Now; while (!mIsCloseAlarm) { count = stream.Read(buffer, 0, mAlarmReadCount); if (count > 0) { strMsg += Encoding.UTF8.GetString(buffer, 0, count); startIndex = strMsg.IndexOf( "<response>" ); endIndex = strMsg.IndexOf( "</response>" ); string xml = strMsg.Substring(startIndex, endIndex - startIndex + "</response>" .Length); res = HuaweiXMLHelper.GetNormalResponse(xml); strMsg = strMsg.Substring(endIndex + "</response>" .Length); startIndex = -1; endIndex = -1; break ; } dtEnd = DateTime.Now; if ((dtEnd - dtStart).TotalSeconds > 10) { throw new Exception( "连接信息未有获取到,需要重启报警!" ); } } while (!mIsCloseAlarm) { count = stream.Read(buffer, 0, mAlarmReadCount); if (count > 0) { string temp = Encoding.UTF8.GetString(buffer, 0, count); strMsg += temp; while (strMsg.Length > 0) { if (startIndex == -1) //未发现第一个<task-info> { startIndex = strMsg.IndexOf( "<task-info>" ); if (startIndex == -1) { if (strMsg.Length >= "<task-info>" .Length) { strMsg = strMsg.Substring(strMsg.Length - "<task-info>" .Length); } break ; } } if (startIndex >= 0) { int i = startIndex + "<task-info>" .Length; int taskInfoEndIndex = strMsg.IndexOf( "</task-info>" , i); if (taskInfoEndIndex > 0) //必须有任务结束节点 { i = taskInfoEndIndex + "</task-info>" .Length; int i1 = strMsg.IndexOf( "</attach-rules>" , i); //找到轨迹节点结束 int i2 = strMsg.IndexOf( "</alarm>" , i); //找到报警节点结束,发现一条报警 if (i1 == -1 && i2 == -1) //没有标志结束 { break ; } else if (i1 >= 0 && (i1 < i2 || i2 == -1)) //找到轨迹结束节点 { strMsg = strMsg.Substring(i1 + "</attach-rules>" .Length); startIndex = -1; endIndex = -1; continue ; } else if (i2 > 0 && (i2 < i1 || i1 == -1)) //找报警节点 { endIndex = i2; //找到报警节点结束,发现一条报警 string alarmXml = "<taskalarm>" + strMsg.Substring(startIndex, endIndex - startIndex + "</alarm>" .Length) + "</taskalarm>" ; Thread th = new Thread( new ThreadStart( delegate () { ParseAlarmXml(alarmXml); })); th.IsBackground = true ; th.Start(); strMsg = strMsg.Substring(endIndex + "</alarm>" .Length); startIndex = -1; endIndex = -1; continue ; } } else { break ; } } } } else { Console.WriteLine( "##########读取报警反馈:无" ); Thread.Sleep(1000); } } } catch (System.Threading.ThreadAbortException ex) { mLog.Info( "AlarmCallBack...7" ); try { if (stream != null ) { stream.Close(); stream.Dispose(); response.Close(); } } catch { } mLog.Info( "AlarmCallBack线程已人为终止!--0" + ex.Message, ex); } catch (IOException ex) { mLog.Info( "AlarmCallBack...8" ); try { if (stream != null ) { stream.Close(); stream.Dispose(); response.Close(); } } catch { } } catch (ObjectDisposedException ex) { mLog.Info( "AlarmCallBack...9" ); mLog.Info( "AlarmCallBack读取流已人为终止!--2" + ex.Message, ex); try { if (stream != null ) { stream.Close(); stream.Dispose(); response.Close(); } } catch { } } catch (System.Exception ex) { mLog.Info( "AlarmCallBack...10" ); mLog.Error( "AlarmCallBack 0:" + ex.Message,ex); try { if (stream != null ) { stream.Close(); stream.Dispose(); response.Close(); } } catch { } } finally { } })); alarmThead[1].IsBackground = true ; alarmThead[1].Start(); } catch (System.Exception ex) { mLog.Info( "AlarmCallBack...11" ); mLog.Error( "AlarmCallBack 1:" + ex.Message,ex); mLog.Info( "AlarmCallBack开始重新报警连接....3" ); mTimes = 50; } finally { } } |
以上就是本文的全部内容,希望对大家的学习有所帮助文章源自设计学徒自学网-https://www.sx1c.com/25860.html 文章源自设计学徒自学网-https://www.sx1c.com/25860.html
继续阅读
我的微信
微信扫一扫
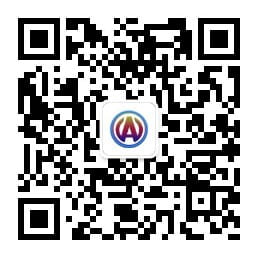
我的微信
惠生活福利社
微信扫一扫
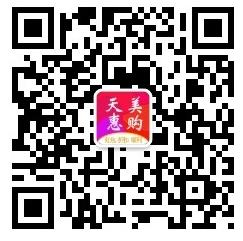
我的公众号
评论