AutoCAD绘图批量打印
from pyautocad import Autocad import win32com.client import win32print import pythoncom import time # 创建AutoCAD对象 acad = win32com.client.Dispatch("AutoCAD.Application.23") # 获取活动文档和模型空间 acaddoc = acad.ActiveDocument acadmod = acaddoc.ModelSpace layout = acaddoc.layouts.item('Model') plot = acaddoc.Plot # 获取默认打印机 _PRINTER = win32print.GetDefaultPrinter() _HPRINTER = win32print.OpenPrinter(_PRINTER) # 打印样式设置函数 def PrinterStyleSetting(): acaddoc.SetVariable('BACKGROUNDPLOT', 0) layout.ConfigName = 'RICOH MP C2011' layout.StyleSheet = 'monochrome.ctb' layout.PlotWithLineweights = False layout.CanonicalMediaName = 'A3' layout.PlotRotation = 1 layout.CenterPlot = True layout.PlotWithPlotStyles = True layout.PlotHidden = False print(layout.GetPlotStyleTableNames()[-1]) layout.PlotType = 4 # 默认起始位置和绘图尺寸 DEFAULT_START_POSITION = (3, 3) DRAWING_SIZE = (598, 422) DRAWING_INTEND = 700 # 后台打印类 class BackPrint(object): _instance = None def __new__(cls, *args, **kw): if cls._instance is None: cls._instance = super(BackPrint, cls).__new__(cls) return cls._instance def __init__(self, PositionX, PositionY): self.x = PositionX self.y = PositionY @staticmethod def APoint(x, y): return win32com.client.VARIANT(pythoncom.VT_ARRAY | pythoncom.VT_R8, (x, y)) def run(self, Scale=1.0): po1 = self.APoint(self.x * Scale - 1, self.y * Scale) po2 = self.APoint(self.x * Scale - 1 + DRAWING_SIZE[0], self.y * Scale + DRAWING_SIZE[1]) layout.SetWindowToPlot(po1, po2) PrinterStyleSetting() plot.PlotToDevice() # 打印任务类 class PrintTask: def __init__(self, maxPrintPositionArray, startPosition=(DEFAULT_START_POSITION[0], DEFAULT_START_POSITION[1])): self._PrinterStatus = 'Waiting' self.maxPrintPositionArray = maxPrintPositionArray self.printBasePointArray = [] self.taskPoint = startPosition self.PrintingTaskNumber = 0 def runtask(self): if not self.printBasePointArray: self.printBasePointArray = self.generalPrintBasePointArray(self.maxPrintPositionArray) for position in self.printBasePointArray: self.taskPoint = position current_task = BackPrint(*position) current_task.run() self.PrintingTaskNumber = len(win32print.EnumJobs(_HPRINTER, 0, -1, 1)) while self.PrintingTaskNumber >= 5: time.sleep(1) self.PrintingTaskNumber = len(win32print.EnumJobs(_HPRINTER, 0, -1, 1)) time.sleep(1) def ResumeTask(self): pass def generalPrintBasePointArray(self, maxPrintPositionArray): printBasePointArray = [] next_drawing_xORy_intend = DRAWING_INTEND current_x = int((self.taskPoint[0] - 4) / DRAWING_INTEND) * DRAWING_INTEND + DEFAULT_START_POSITION[0] current_y = int((self.taskPoint[1] - 4) / DRAWING_INTEND) * DRAWING_INTEND + DEFAULT_START_POSITION[1] for position in maxPrintPositionArray: while current_x <= position + DEFAULT_START_POSITION[0]: printBasePointArray.append((current_x, current_y)) current_x += next_drawing_xORy_intend current_x = DEFAULT_START_POSITION[0] current_y += next_drawing_xORy_intend return printBasePointArray def getTaskNumber(self): TaskNumber = self.PrintingTaskNumber try: TaskNumber = len(win32print.EnumJobs(_HPRINTER, 0, -1, 1)) return TaskNumber except Exception as e: return TaskNumber if __name__ == '__main__': task = PrintTask([27895, ], (6194, 4)) task.runtask()文章源自设计学徒自学网-https://www.sx1c.com/46674.html文章源自设计学徒自学网-https://www.sx1c.com/46674.html
继续阅读
我的微信
微信扫一扫
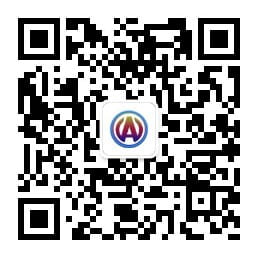
我的微信
惠生活福利社
微信扫一扫
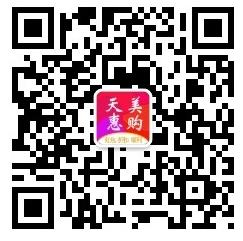
我的公众号
评论